A virtualized cluster-based compute service. More...
#include <VirtualizedClusterComputeService.h>
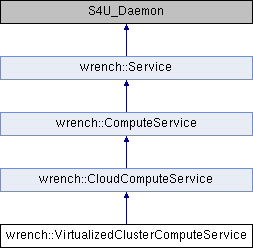
Public Member Functions | |
VirtualizedClusterComputeService (const std::string &hostname, std::vector< std::string > &execution_hosts, std::string scratch_space_mount_point, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) | |
Constructor. More... | |
virtual void | migrateVM (const std::string &vm_hostname, const std::string &dest_pm_hostname) |
Synchronously migrate a VM to another physical host. More... | |
virtual std::shared_ptr< BareMetalComputeService > | startVM (const std::string &vm_name) |
Start a VM. More... | |
virtual std::shared_ptr< BareMetalComputeService > | startVM (const std::string &vm_name, const std::string &pm_name) |
Start a VM. More... | |
![]() | |
CloudComputeService (const std::string &hostname, std::vector< std::string > &execution_hosts, std::string scratch_space_mount_point, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) | |
Constructor. More... | |
virtual std::string | createVM (unsigned long num_cores, double ram_memory, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) |
Create a BareMetalComputeService VM (balances load on execution hosts) More... | |
virtual std::string | createVM (unsigned long num_cores, double ram_memory, std::string desired_vm_name, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) |
Create a BareMetalComputeService VM (balances load on execution hosts) More... | |
virtual void | destroyVM (const std::string &vm_name) |
Destroy a VM. More... | |
std::vector< std::string > | getExecutionHosts () |
Get the list of execution hosts available to run VMs. More... | |
virtual bool | isVMDown (const std::string &vm_name) |
Method to check whether a VM is currently down. More... | |
virtual bool | isVMRunning (const std::string &vm_name) |
Method to check whether a VM is currently running. More... | |
virtual bool | isVMSuspended (const std::string &vm_name) |
Method to check whether a VM is currently running. More... | |
virtual void | resumeVM (const std::string &vm_name) |
Resume a suspended VM. More... | |
virtual void | shutdownVM (const std::string &vm_name) |
Shutdown an active VM. More... | |
virtual std::shared_ptr< BareMetalComputeService > | startVM (const std::string &vm_name) |
Start a VM. More... | |
virtual void | suspendVM (const std::string &vm_name) |
Suspend a running VM. More... | |
![]() | |
std::map< std::string, double > | getCoreFlopRate () |
Get the per-core flop rate of the compute service's hosts. More... | |
double | getFreeScratchSpaceSize () |
Get the free space on the compute service's scratch storage space. More... | |
std::map< std::string, double > | getMemoryCapacity () |
Get the RAM capacities for each of the compute service's hosts. More... | |
unsigned long | getNumHosts () |
Get the number of hosts that the compute service manages. More... | |
std::map< std::string, double > | getPerHostAvailableMemoryCapacity () |
Get ram availability for each of the compute service's host. More... | |
std::map< std::string, unsigned long > | getPerHostNumCores () |
Get core counts for each of the compute service's host. More... | |
std::map< std::string, unsigned long > | getPerHostNumIdleCores () |
Get idle core counts for each of the compute service's host. More... | |
std::shared_ptr< StorageService > | getScratchSharedPtr () |
Get a shared pointer to the compute service's scratch storage space. More... | |
unsigned long | getTotalNumCores () |
Get the total core counts for all hosts of the compute service. More... | |
virtual unsigned long | getTotalNumIdleCores () |
Get the total idle core count for all hosts of the compute service. More... | |
double | getTotalScratchSpaceSize () |
Get the total capacity of the compute service's scratch storage space. More... | |
double | getTTL () |
Get the time-to-live of the compute service. More... | |
bool | hasScratch () |
Checks if the compute service has a scratch space. More... | |
void | stop () override |
Stop the compute service - must be called by the stop() method of derived classes. | |
void | submitJob (WorkflowJob *job, std::map< std::string, std::string >={}) |
Submit a job to the compute service. More... | |
bool | supportsPilotJobs () |
Get whether the compute service supports pilot jobs or not. More... | |
bool | supportsStandardJobs () |
Get whether the compute service supports standard jobs or not. More... | |
void | terminateJob (WorkflowJob *job) |
Terminate a previously-submitted job (which may or may not be running yet) More... | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
void | suspend () |
Suspend the service. | |
Additional Inherited Members | |
![]() | |
static constexpr unsigned long | ALL_CORES = ULONG_MAX |
A convenient constant to mean "use all cores of a physical host" whenever a number of cores is needed when instantiating compute services. | |
static constexpr double | ALL_RAM = DBL_MAX |
A convenient constant to mean "use all ram of a physical host" whenever a ram capacity is needed when instantiating compute services. | |
![]() | |
std::vector< std::string > | execution_hosts |
List of execution host names. | |
std::map< std::string, unsigned long > | used_cores_per_execution_host |
Map of number of used cores at the hosts. | |
std::map< std::string, double > | used_ram_per_execution_host |
Map of used RAM at the hosts. | |
std::map< std::string, std::pair< std::shared_ptr< S4U_VirtualMachine >, std::shared_ptr< BareMetalComputeService > > > | vm_list |
A map of VMs. | |
Detailed Description
A virtualized cluster-based compute service.
Constructor & Destructor Documentation
◆ VirtualizedClusterComputeService()
wrench::VirtualizedClusterComputeService::VirtualizedClusterComputeService | ( | const std::string & | hostname, |
std::vector< std::string > & | execution_hosts, | ||
std::string | scratch_space_mount_point, | ||
std::map< std::string, std::string > | property_list = {} , |
||
std::map< std::string, double > | messagepayload_list = {} |
||
) |
Constructor.
- Parameters
-
hostname the name of the hostcreate on which to start the service execution_hosts the hosts available for running virtual machines scratch_space_mount_point the mount of of the scratch space of the cloud service ("" means none) property_list a property list ({} means "use all defaults") messagepayload_list a message payload list ({} means "use all defaults")
- Exceptions
-
std::runtime_error
Member Function Documentation
◆ migrateVM()
|
virtual |
Synchronously migrate a VM to another physical host.
- Parameters
-
vm_name virtual machine hostname dest_pm_hostname the name of the destination physical machine host
- Exceptions
-
std::invalid_argument
◆ startVM() [1/2]
std::shared_ptr< BareMetalComputeService > wrench::CloudComputeService::startVM |
Start a VM.
- Parameters
-
vm_name the name of the VM
- Returns
- A BareMetalComputeService that runs on the VM
- Exceptions
-
WorkflowExecutionException std::invalid_argument
◆ startVM() [2/2]
|
virtual |
Start a VM.
- Parameters
-
vm_name the name of the VM pm_name the physical host on which to start the VM
- Returns
- The compute service running on the VM
- Exceptions
-
WorkflowExecutionException std::invalid_argument
The documentation for this class was generated from the following files:
- VirtualizedClusterComputeService.h
- VirtualizedClusterComputeService.cpp