A storage service that provides direct access to some storage resources (e.g., one or more disks). An important (configurable) property of the storage service is SimpleStorageServiceProperty::BUFFER_SIZE (see documentation thereof), which defines the buffer size that the storage service uses. Specifically, when the storage service receives/sends data from/to the network, it does so in a loop over data "chunks", with pipelined network and disk I/O operations. The smaller the buffer size the more "fluid" the model, but the more time-consuming the simulation. A large buffer size, however, may lead to less realistic simulations. At the extreme, an infinite buffer size would correspond to fully sequential executions (first a network receive/send, and then a disk write/read). Setting the buffer size to "0" corresponds to a fully fluid model in which individual data chunk operations are not simulated, thus achieving both accuracy (unless one specifically wishes to study the effects of buffering) and quick simulation times. For now, setting the buffer size to "0" is not implemented. The default buffer size is 10 MiB (note that the user can always declare a disk with arbitrary bandwidth in the platform description XML). More...
#include <SimpleStorageService.h>
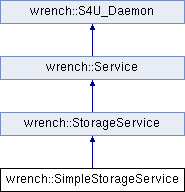
Public Member Functions | |
SimpleStorageService (std::string hostname, std::set< std::string > mount_points, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) | |
Public constructor. More... | |
~SimpleStorageService () override | |
Destructor. | |
void | cleanup (bool has_returned_from_main, int return_value) override |
Cleanup method. More... | |
![]() | |
StorageService (const std::string &hostname, const std::set< std::string > mount_points, const std::string &service_name, const std::string &data_mailbox_name_prefix) | |
Constructor. More... | |
virtual std::map< std::string, double > | getFreeSpace () |
Synchronously asks the storage service for its capacity at all its mount points. More... | |
std::string | getMountPoint () |
Get the mount point (will throw is more than one) More... | |
std::set< std::string > | getMountPoints () |
Get the set of mount points. More... | |
virtual std::map< std::string, double > | getTotalSpace () |
Get the total static capacity of the storage service (in zero simulation time) More... | |
bool | hasMountPoint (std::string mp) |
Checked whether the storage service has a particular mount point. More... | |
bool | hasMultipleMountPoints () |
Checked whether the storage service has multiple mount points. More... | |
bool | isScratch () |
Determines whether the storage service is a scratch service of a ComputeService. More... | |
void | setScratch () |
Indicate that this storace service is a scratch service of a ComputeService. | |
void | stop () override |
Stop the service. | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getMessagePayloadValue (std::string) |
Get a message payload of the Service as a double. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | setStateToDown () |
Set the state of the service to DOWN. | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
void | suspend () |
Suspend the service. | |
![]() | |
S4U_Daemon (std::string hostname, std::string process_name_prefix, std::string mailbox_prefix) | |
Constructor (daemon with a mailbox) More... | |
virtual | ~S4U_Daemon () |
void | createLifeSaver (std::shared_ptr< S4U_Daemon > reference) |
Create a life saver for the daemon. More... | |
std::string | getName () |
Retrieve the process name. More... | |
int | getReturnValue () |
Returns the value returned by main() (if the daemon has returned from main) More... | |
S4U_Daemon::State | getState () |
Get the daemon's state. More... | |
bool | hasReturnedFromMain () |
Returns true if the daemon has returned from main() (i.e., not brutally killed) More... | |
bool | isDaemonized () |
Return the daemonized status of the daemon. More... | |
bool | isSetToAutoRestart () |
Return the auto-restart status of the daemon. More... | |
std::pair< bool, int > | join () |
Join (i.e., wait for) the daemon. More... | |
void | resumeActor () |
Resume the daemon/actor. | |
void | setupOnExitFunction () |
Sets up the on_exit functionf for the actor. | |
void | startDaemon (bool daemonized, bool auto_restart) |
Start the daemon. More... | |
void | suspendActor () |
Suspend the daemon/actor. | |
Additional Inherited Members | |
![]() | |
enum | State { UP, DOWN, SUSPENDED } |
Daemon states. More... | |
![]() | |
static void | copyFile (WorkflowFile *file, std::shared_ptr< FileLocation > src_location, std::shared_ptr< FileLocation > dst_location) |
Synchronously ask the storage service to read a file from another storage service. More... | |
static void | deleteFile (WorkflowFile *file, std::shared_ptr< FileLocation > location, std::shared_ptr< FileRegistryService > file_registry_service=nullptr) |
Synchronously delete a file at a location. More... | |
static void | initiateFileCopy (std::string answer_mailbox, WorkflowFile *file, std::shared_ptr< FileLocation > src_location, std::shared_ptr< FileLocation > dst_location) |
Asynchronously ask for a file copy between two storage services. More... | |
static bool | lookupFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Synchronously asks the storage service whether it holds a file. More... | |
static void | readFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Synchronously read a file from the storage service. More... | |
static void | readFiles (std::map< WorkflowFile *, std::shared_ptr< FileLocation >> locations) |
Synchronously and sequentially read a set of files from storage services. More... | |
static void | writeFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Synchronously write a file to the storage service. More... | |
static void | writeFiles (std::map< WorkflowFile *, std::shared_ptr< FileLocation >> locations) |
Synchronously and sequentially upload a set of files from storage services. More... | |
![]() | |
static void | cleanupTrackedServices () |
Go through the tracked services and remove all entries with a refcount of 1! | |
static void | clearTrackedServices () |
Forget all tracked services. | |
static void | increaseNumCompletedServicesCount () |
Increase the completed service count. | |
![]() | |
std::string | hostname |
The name of the host on which the daemon is running. | |
std::string | initial_mailbox_name |
The initial name of the daemon's mailbox. | |
LifeSaver * | life_saver = nullptr |
The daemon's life saver. | |
std::string | mailbox_name |
The current name of the daemon's mailbox. | |
std::string | process_name |
The name of the daemon. | |
Simulation * | simulation |
a pointer to the simulation object | |
![]() | |
Service (std::string hostname, std::string process_name_prefix, std::string mailbox_name_prefix) | |
Constructor. More... | |
~Service () | |
Destructor. | |
template<class T > | |
std::shared_ptr< T > | getSharedPtr () |
Method to retrieve the shared_ptr to a service. More... | |
void | serviceSanityCheck () |
Check whether the service is properly configured and running. More... | |
void | setMessagePayload (std::string, double) |
Set a message payload of the Service. More... | |
void | setMessagePayloads (std::map< std::string, double > default_messagepayload_values, std::map< std::string, double > overriden_messagepayload_values) |
Set default and user-defined message payloads. More... | |
void | setProperties (std::map< std::string, std::string > default_property_values, std::map< std::string, std::string > overriden_property_values) |
Set default and user-defined properties. More... | |
void | setProperty (std::string, std::string) |
Set a property of the Service. More... | |
![]() | |
void | acquireDaemonLock () |
Lock the daemon's lock. | |
void | killActor () |
Kill the daemon/actor (does nothing if already dead) More... | |
void | releaseDaemonLock () |
Unlock the daemon's lock. | |
void | runMainMethod () |
Method that run's the user-defined main method (that's called by the S4U actor class) | |
![]() | |
static void | stageFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Store a file at a particular mount point BEFORE the simulation is launched. More... | |
![]() | |
static void | assertServiceIsUp (std::shared_ptr< Service > s) |
Assert for the service being up. More... | |
template<class T > | |
static std::shared_ptr< T > | getServiceByName (std::string name) |
Method to retrieve the shared_ptr to a service based on the service's name (not efficient) More... | |
![]() | |
unsigned long | buffer_size |
The service's buffer size. | |
std::map< std::string, std::unique_ptr< LogicalFileSystem > > | file_systems |
File systems. | |
![]() | |
std::map< std::string, double > | messagepayload_list |
The service's messagepayload list. | |
std::string | name |
The service's name. | |
double | network_timeout = 30.0 |
The time (in seconds) after which a service that doesn't send back a reply (control) message causes a NetworkTimeOut exception. (default: 30 second; if <0 never timeout) | |
std::map< std::string, std::string > | property_list |
The service's property list. | |
![]() | |
unsigned int | num_starts = 0 |
The number of time that this daemon has started (i.e., 1 + number of restarts) | |
State | state |
The service's state. | |
Detailed Description
A storage service that provides direct access to some storage resources (e.g., one or more disks). An important (configurable) property of the storage service is SimpleStorageServiceProperty::BUFFER_SIZE (see documentation thereof), which defines the buffer size that the storage service uses. Specifically, when the storage service receives/sends data from/to the network, it does so in a loop over data "chunks", with pipelined network and disk I/O operations. The smaller the buffer size the more "fluid" the model, but the more time-consuming the simulation. A large buffer size, however, may lead to less realistic simulations. At the extreme, an infinite buffer size would correspond to fully sequential executions (first a network receive/send, and then a disk write/read). Setting the buffer size to "0" corresponds to a fully fluid model in which individual data chunk operations are not simulated, thus achieving both accuracy (unless one specifically wishes to study the effects of buffering) and quick simulation times. For now, setting the buffer size to "0" is not implemented. The default buffer size is 10 MiB (note that the user can always declare a disk with arbitrary bandwidth in the platform description XML).
Constructor & Destructor Documentation
◆ SimpleStorageService()
wrench::SimpleStorageService::SimpleStorageService | ( | std::string | hostname, |
std::set< std::string > | mount_points, | ||
std::map< std::string, std::string > | property_list = {} , |
||
std::map< std::string, double > | messagepayload_list = {} |
||
) |
Public constructor.
- Parameters
-
hostname the name of the host on which to start the service mount_points the set of mount points property_list a property list ({} means "use all defaults") messagepayload_list a message payload list ({} means "use all defaults")
Member Function Documentation
◆ cleanup()
|
overridevirtual |
Cleanup method.
- Parameters
-
has_returned_from_main whether main() returned return_value the return value (if main() returned)
Reimplemented from wrench::S4U_Daemon.
The documentation for this class was generated from the following files:
- SimpleStorageService.h
- SimpleStorageService.cpp