A network proximity service that continuously estimates inter-host latencies and can be queried for such estimates. More...
#include <NetworkProximityService.h>
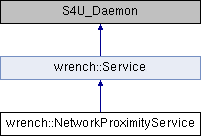
Public Member Functions | |
NetworkProximityService (std::string db_hostname, std::vector< std::string > hosts_in_network, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) | |
Constructor. More... | |
std::pair< std::pair< double, double >, double > | getHostCoordinate (std::string) |
Look up the current (x,y) coordinates of a given host (only for a Vivaldi network service type) More... | |
std::vector< std::string > | getHostnameList () |
Gets the list of hosts monitored by this service (does not involve simulated network communications with the service) More... | |
std::pair< double, double > | getHostPairDistance (std::pair< std::string, std::string > hosts) |
Look up a proximity value in database. More... | |
std::string | getNetworkProximityServiceType () |
Get the network proximity service type. More... | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
virtual void | stop () |
Synchronously stop the service (does nothing if the service is already stopped) More... | |
void | suspend () |
Suspend the service. | |
Detailed Description
A network proximity service that continuously estimates inter-host latencies and can be queried for such estimates.
Constructor & Destructor Documentation
◆ NetworkProximityService()
wrench::NetworkProximityService::NetworkProximityService | ( | std::string | hostname, |
std::vector< std::string > | hosts_in_network, | ||
std::map< std::string, std::string > | property_list = {} , |
||
std::map< std::string, double > | messagepayload_list = {} |
||
) |
Constructor.
- Parameters
-
hostname the name of the host on which to start the service hosts_in_network the hosts participating in network measurements property_list a property list ({} means "use all defaults") messagepayload_list a message payload list ({} means "use all defaults")
Member Function Documentation
◆ getHostCoordinate()
std::pair< std::pair< double, double >, double > wrench::NetworkProximityService::getHostCoordinate | ( | std::string | requested_host | ) |
Look up the current (x,y) coordinates of a given host (only for a Vivaldi network service type)
- Parameters
-
requested_host the host whose coordinates are being requested
- Returns
- A pair:
- a (x,y) coordinate pair
- a timestamp (the oldest timestamp of measurements usead to compute the coordinate)
- Exceptions
-
WorkFlowExecutionException std::runtime_error
◆ getHostnameList()
std::vector< std::string > wrench::NetworkProximityService::getHostnameList | ( | ) |
Gets the list of hosts monitored by this service (does not involve simulated network communications with the service)
- Returns
- a list of hostnames
◆ getHostPairDistance()
std::pair< double, double > wrench::NetworkProximityService::getHostPairDistance | ( | std::pair< std::string, std::string > | hosts | ) |
Look up a proximity value in database.
- Parameters
-
hosts the pair of hosts whose proximity is of interest
- Returns
- A pair:
- The proximity value between the pair of hosts (or DBL_MAX if none)
- The timestamp of the oldest measurement use to compute the proximity value (or -1.0 if none)
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getNetworkProximityServiceType()
std::string wrench::NetworkProximityService::getNetworkProximityServiceType | ( | ) |
Get the network proximity service type.
- Returns
- a string specifying the network proximity service type
The documentation for this class was generated from the following files:
- NetworkProximityService.h
- NetworkProximityService.cpp