The storage service base class. More...
#include <StorageService.h>
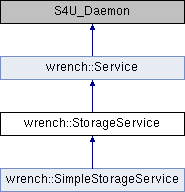
Public Member Functions | |
virtual std::map< std::string, double > | getFreeSpace () |
Synchronously asks the storage service for its capacity at all its mount points. More... | |
std::string | getMountPoint () |
Get the mount point (will throw is more than one) More... | |
std::set< std::string > | getMountPoints () |
Get the set of mount points. More... | |
virtual std::map< std::string, double > | getTotalSpace () |
Get the total static capacity of the storage service (in zero simulation time) More... | |
bool | hasMountPoint (std::string mp) |
Checked whether the storage service has a particular mount point. More... | |
bool | hasMultipleMountPoints () |
Checked whether the storage service has multiple mount points. More... | |
void | stop () override |
Stop the service. | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
void | suspend () |
Suspend the service. | |
Static Public Member Functions | |
static void | deleteFile (WorkflowFile *file, std::shared_ptr< FileLocation > location, std::shared_ptr< FileRegistryService > file_registry_service=nullptr) |
Synchronously delete a file at a location. More... | |
static bool | lookupFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Synchronously asks the storage service whether it holds a file. More... | |
static void | readFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Synchronously read a file from the storage service. More... | |
static void | writeFile (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Synchronously write a file to the storage service. More... | |
Detailed Description
The storage service base class.
Member Function Documentation
◆ deleteFile()
|
static |
Synchronously delete a file at a location.
- Parameters
-
file the file location the file's location file_registry_service a file registry service that should be updated once the file deletion has (successfully) completed (none if nullptr)
- Exceptions
-
WorkflowExecutionException std::runtime_error std::invalid_argument
◆ getFreeSpace()
|
virtual |
Synchronously asks the storage service for its capacity at all its mount points.
- Returns
- The free space in bytes of each mount point, as a map
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getMountPoint()
std::string wrench::StorageService::getMountPoint | ( | ) |
Get the mount point (will throw is more than one)
- Returns
- the (sole) mount point of the service
◆ getMountPoints()
std::set< std::string > wrench::StorageService::getMountPoints | ( | ) |
Get the set of mount points.
- Returns
- the set of mount points
◆ getTotalSpace()
|
virtual |
Get the total static capacity of the storage service (in zero simulation time)
- Returns
- capacity of the storage service (double) for each mount point, in a map
◆ hasMountPoint()
bool wrench::StorageService::hasMountPoint | ( | std::string | mp | ) |
Checked whether the storage service has a particular mount point.
- Parameters
-
mp a mount point
- Returns
- true whether the service has that mount point
◆ hasMultipleMountPoints()
bool wrench::StorageService::hasMultipleMountPoints | ( | ) |
Checked whether the storage service has multiple mount points.
- Returns
- true whether the service has multiple mount points
◆ lookupFile()
|
static |
Synchronously asks the storage service whether it holds a file.
- Parameters
-
file the file location the file location
- Returns
- true or false
- Exceptions
-
WorkflowExecutionException std::invalid_arguments
◆ readFile()
|
static |
Synchronously read a file from the storage service.
- Parameters
-
file the file location the location to read the file from
- Exceptions
-
WorkflowExecutionException std::invalid_arguments
◆ writeFile()
|
static |
Synchronously write a file to the storage service.
- Parameters
-
file the file location the location to write it to
- Exceptions
-
WorkflowExecutionException
The documentation for this class was generated from the following files:
- StorageService.h
- StorageService.cpp