A standard (i.e., non-pilot) workflow job that can be submitted to a ComputeService by a WMS (via a JobManager) More...
#include <StandardJob.h>
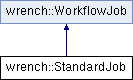
Public Types | |
enum | State { NOT_SUBMITTED, PENDING, RUNNING, COMPLETED, FAILED, TERMINATED } |
Standad job states. More... | |
Public Member Functions | |
std::map< WorkflowFile *, std::shared_ptr< FileLocation > > | getFileLocations () |
Get the file location map for the job. More... | |
unsigned long | getMinimumRequiredMemory () |
Returns the minimum RAM capacity required to run the job (i.e., at least one task in the job cannot run if less ram than this minimum is available) More... | |
unsigned long | getMinimumRequiredNumCores () |
Returns the minimum number of cores required to run the job (i.e., at least one task in the job cannot run if fewer cores than this minimum are available) More... | |
unsigned long | getNumCompletedTasks () |
Get the number of completed tasks in the job. More... | |
unsigned long | getNumTasks () |
Get the number of tasks in the job. More... | |
double | getPostJobOverheadInSeconds () |
get the job's post-overhead More... | |
double | getPreJobOverheadInSeconds () |
get the job's pre-overhead More... | |
unsigned long | getPriority () |
Get the workflow priority value (the maximum priority from all tasks) More... | |
StandardJob::State | getState () |
Get the state of the standard job. More... | |
std::vector< WorkflowTask * > | getTasks () |
Get the workflow tasks in the job. More... | |
void | setPostJobOverheadInSeconds (double overhead) |
sets the job's post-overhead More... | |
void | setPreJobOverheadInSeconds (double overhead) |
sets the job's pre-overhead More... | |
![]() | |
virtual | ~WorkflowJob () |
Destructor. | |
std::string | getCallbackMailbox () |
Get the "next" callback mailbox (returns the origin (i.e., workflow) mailbox if the mailbox stack is empty) More... | |
std::string | getName () |
Get the job's name. More... | |
std::string | getOriginCallbackMailbox () |
Get the "origin" callback mailbox. More... | |
std::shared_ptr< ComputeService > | getParentComputeService () |
Get the parent compute service of the job. More... | |
std::map< std::string, std::string > | getServiceSpecificArguments () |
Return the service-specific arguments that were used during job submission. More... | |
double | getSubmitDate () |
Get the date at which the job was last submitted (<0 means "never submitted") More... | |
std::string | popCallbackMailbox () |
Get the "next" callback mailbox (returns the workflow mailbox if the mailbox stack is empty), and pops it. More... | |
void | pushCallbackMailbox (std::string) |
Pushes a callback mailbox. More... | |
void | setParentComputeService (std::shared_ptr< ComputeService > compute_service) |
Set the parent compute service of the job. More... | |
Public Attributes | |
std::vector< std::tuple< WorkflowFile *, std::shared_ptr< FileLocation > > > | cleanup_file_deletions |
The ordered file deletion operations to perform at the end. | |
std::map< WorkflowFile *, std::shared_ptr< FileLocation > > | file_locations |
The file locations that tasks should read/write files from/to. | |
unsigned long | num_completed_tasks |
The number of computational tasks that have completed. | |
std::vector< std::tuple< WorkflowFile *, std::shared_ptr< FileLocation >, std::shared_ptr< FileLocation > > > | post_file_copies |
The ordered file copy operations to perform after computational tasks. | |
std::vector< std::tuple< WorkflowFile *, std::shared_ptr< FileLocation >, std::shared_ptr< FileLocation > > > | pre_file_copies |
The ordered file copy operations to perform before computational tasks. | |
std::vector< WorkflowTask * > | tasks |
The job's computational tasks. | |
double | total_flops |
The job's total computational cost (in flops) | |
Additional Inherited Members | |
![]() | |
WorkflowJob () | |
Constructor. More... | |
unsigned long | getNewUniqueNumber () |
Generate a unique number (for each newly generated job) More... | |
![]() | |
std::stack< std::string > | callback_mailbox_stack |
Stack of callback mailboxes (to pop notifications) | |
std::string | name |
The job's name. | |
std::shared_ptr< ComputeService > | parent_compute_service |
The compute service to which the job was submitted. | |
std::map< std::string, std::string > | service_specific_args |
Service-specific arguments used during job submission. | |
double | submit_date |
The date at which the job was last submitted. | |
Workflow * | workflow |
The workflow this job belong to. | |
Detailed Description
A standard (i.e., non-pilot) workflow job that can be submitted to a ComputeService by a WMS (via a JobManager)
Member Enumeration Documentation
◆ State
Member Function Documentation
◆ getFileLocations()
std::map< WorkflowFile *, std::shared_ptr< FileLocation > > wrench::StandardJob::getFileLocations | ( | ) |
Get the file location map for the job.
- Returns
- a map of files to storage services
◆ getMinimumRequiredMemory()
unsigned long wrench::StandardJob::getMinimumRequiredMemory | ( | ) |
Returns the minimum RAM capacity required to run the job (i.e., at least one task in the job cannot run if less ram than this minimum is available)
- Returns
- the number of cores
◆ getMinimumRequiredNumCores()
unsigned long wrench::StandardJob::getMinimumRequiredNumCores | ( | ) |
Returns the minimum number of cores required to run the job (i.e., at least one task in the job cannot run if fewer cores than this minimum are available)
- Returns
- the number of cores
◆ getNumCompletedTasks()
unsigned long wrench::StandardJob::getNumCompletedTasks | ( | ) |
Get the number of completed tasks in the job.
- Returns
- the number of completed tasks
◆ getNumTasks()
unsigned long wrench::StandardJob::getNumTasks | ( | ) |
Get the number of tasks in the job.
- Returns
- the number of tasks
◆ getPostJobOverheadInSeconds()
double wrench::StandardJob::getPostJobOverheadInSeconds | ( | ) |
get the job's post-overhead
- Returns
- a number o seconds
◆ getPreJobOverheadInSeconds()
double wrench::StandardJob::getPreJobOverheadInSeconds | ( | ) |
get the job's pre-overhead
- Returns
- a number o seconds
◆ getPriority()
|
virtual |
Get the workflow priority value (the maximum priority from all tasks)
- Returns
- the job priority value
Reimplemented from wrench::WorkflowJob.
◆ getState()
StandardJob::State wrench::StandardJob::getState | ( | ) |
Get the state of the standard job.
- Returns
- the state
◆ getTasks()
std::vector< WorkflowTask * > wrench::StandardJob::getTasks | ( | ) |
Get the workflow tasks in the job.
- Returns
- a vector of workflow tasks
◆ setPostJobOverheadInSeconds()
void wrench::StandardJob::setPostJobOverheadInSeconds | ( | double | overhead | ) |
sets the job's post-overhead
- Parameters
-
overhead the overhead in seconds
◆ setPreJobOverheadInSeconds()
void wrench::StandardJob::setPreJobOverheadInSeconds | ( | double | overhead | ) |
sets the job's pre-overhead
- Parameters
-
overhead the overhead in seconds
The documentation for this class was generated from the following files:
- StandardJob.h
- StandardJob.cpp