A file registry service (a.k.a. replica catalog) that holds a database of which files are available at which storage services. More specifically, the database holds a set of <file, storage service> entries. A WMS can add, lookup, and remove entries at will from this database. More...
#include <FileRegistryService.h>
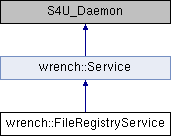
Public Member Functions | |
FileRegistryService (std::string hostname, std::map< std::string, std::string > property_list={}, std::map< std::string, double > messagepayload_list={}) | |
Constructor. More... | |
void | addEntry (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Add an entry. More... | |
std::set< std::shared_ptr< FileLocation > > | lookupEntry (WorkflowFile *file) |
Lookup entries for a file. More... | |
std::map< double, std::shared_ptr< FileLocation > > | lookupEntry (WorkflowFile *file, std::string reference_host, std::shared_ptr< NetworkProximityService > network_proximity_service) |
Lookup entries for a file, including for each entry a network distance from a reference host (as determined by a network proximity service) More... | |
void | removeEntry (WorkflowFile *file, std::shared_ptr< FileLocation > location) |
Remove an entry. More... | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
virtual void | stop () |
Synchronously stop the service (does nothing if the service is already stopped) More... | |
void | suspend () |
Suspend the service. | |
Detailed Description
A file registry service (a.k.a. replica catalog) that holds a database of which files are available at which storage services. More specifically, the database holds a set of <file, storage service> entries. A WMS can add, lookup, and remove entries at will from this database.
Constructor & Destructor Documentation
◆ FileRegistryService()
|
explicit |
Constructor.
- Parameters
-
hostname the hostname on which to start the service property_list a property list ({} means "use all defaults") messagepayload_list a message payload list ({} means "use all defaults")
Member Function Documentation
◆ addEntry()
void wrench::FileRegistryService::addEntry | ( | WorkflowFile * | file, |
std::shared_ptr< FileLocation > | location | ||
) |
Add an entry.
- Parameters
-
file a file location a file location
- Exceptions
-
WorkflowExecutionException std::invalid_argument std::runtime_error
◆ lookupEntry() [1/2]
std::set< std::shared_ptr< FileLocation > > wrench::FileRegistryService::lookupEntry | ( | WorkflowFile * | file | ) |
Lookup entries for a file.
- Parameters
-
file the file to lookup
- Returns
- The list locations for the file
- Exceptions
-
WorkflowExecutionException std::invalid_argument std::runtime_error
◆ lookupEntry() [2/2]
std::map< double, std::shared_ptr< FileLocation > > wrench::FileRegistryService::lookupEntry | ( | WorkflowFile * | file, |
std::string | reference_host, | ||
std::shared_ptr< NetworkProximityService > | network_proximity_service | ||
) |
Lookup entries for a file, including for each entry a network distance from a reference host (as determined by a network proximity service)
- Parameters
-
file the file to lookup reference_host reference host from which network proximity values are to be measured network_proximity_service the network proximity service to use
- Returns
- a map of <distance , file location> pairs
◆ removeEntry()
void wrench::FileRegistryService::removeEntry | ( | WorkflowFile * | file, |
std::shared_ptr< FileLocation > | location | ||
) |
Remove an entry.
- Parameters
-
file a file location a file location
- Exceptions
-
WorkflowExecutionException std::invalid_argument std::runtime_error
The documentation for this class was generated from the following files:
- FileRegistryService.h
- FileRegistryService.cpp