A workflow management system (WMS) More...
#include <WMS.h>
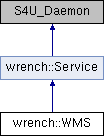
Public Member Functions | |
void | addDynamicOptimization (std::unique_ptr< DynamicOptimization >) |
Add a dynamic optimization to the list of optimizations. Optimizations are executed in order of insertion. More... | |
void | addStaticOptimization (std::unique_ptr< StaticOptimization >) |
Add a static optimization to the list of optimizations. Optimizations are executed in order of insertion. More... | |
void | addWorkflow (Workflow *workflow, double start_time=0) |
Assign a workflow to the WMS. More... | |
PilotJobScheduler * | getPilotJobScheduler () |
Get the WMS's pilot scheduler. More... | |
StandardJobScheduler * | getStandardJobScheduler () |
Get the WMS's pilot scheduler. More... | |
Workflow * | getWorkflow () |
Get the workflow that was assigned to the WMS. More... | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
virtual void | stop () |
Synchronously stop the service (does nothing if the service is already stopped) More... | |
void | suspend () |
Suspend the service. | |
Protected Member Functions | |
WMS (std::unique_ptr< StandardJobScheduler > standard_job_scheduler, std::unique_ptr< PilotJobScheduler > pilot_job_scheduler, const std::set< std::shared_ptr< ComputeService >> &compute_services, const std::set< std::shared_ptr< StorageService >> &storage_services, const std::set< std::shared_ptr< NetworkProximityService >> &network_proximity_services, std::shared_ptr< FileRegistryService > file_registry_service, const std::string &hostname, const std::string suffix) | |
Constructor: a WMS with a workflow instance, a scheduler implementation, and a list of compute services. More... | |
void | checkDeferredStart () |
Check whether the WMS has a deferred start simulation time (likely the first call in the main() routine of any WMS. More... | |
std::shared_ptr< DataMovementManager > | createDataMovementManager () |
Instantiate and start a data movement manager. More... | |
std::shared_ptr< EnergyMeterService > | createEnergyMeter (const std::map< std::string, double > &measurement_periods) |
Instantiate and start an energy meter. More... | |
std::shared_ptr< EnergyMeterService > | createEnergyMeter (const std::vector< std::string > &hostnames, double measurement_period) |
Instantiate and start an energy meter. More... | |
std::shared_ptr< JobManager > | createJobManager () |
Instantiate and start a job manager. More... | |
template<class T > | |
std::set< std::shared_ptr< T > > | getAvailableComputeServices () |
Obtain the list of compute services available to the WMS. More... | |
std::shared_ptr< FileRegistryService > | getAvailableFileRegistryService () |
Obtain the file registry service available to the WMS. More... | |
std::set< std::shared_ptr< NetworkProximityService > > | getAvailableNetworkProximityServices () |
Obtain the list of network proximity services available to the WMS. More... | |
std::set< std::shared_ptr< StorageService > > | getAvailableStorageServices () |
Obtain the list of storage services available to the WMS. More... | |
virtual void | processEventFileCopyCompletion (std::shared_ptr< FileCopyCompletedEvent >) |
Process a file copy completion event. More... | |
virtual void | processEventFileCopyFailure (std::shared_ptr< FileCopyFailedEvent >) |
Process a file copy failure event. More... | |
virtual void | processEventPilotJobExpiration (std::shared_ptr< PilotJobExpiredEvent >) |
Process a pilot job expiration event. More... | |
virtual void | processEventPilotJobStart (std::shared_ptr< PilotJobStartedEvent >) |
Process a pilot job start event. More... | |
virtual void | processEventStandardJobCompletion (std::shared_ptr< StandardJobCompletedEvent >) |
Process a standard job completion event. More... | |
virtual void | processEventStandardJobFailure (std::shared_ptr< StandardJobFailedEvent >) |
Process a standard job failure event. More... | |
virtual void | processEventTimer (std::shared_ptr< TimerEvent >) |
Process a timer event. More... | |
void | runDynamicOptimizations () |
Perform dynamic optimizations. Optimizations are executed in order of insertion. | |
void | runStaticOptimizations () |
Perform static optimizations. Optimizations are executed in order of insertion. | |
void | setTimer (double date, std::string message) |
Sets a timer (which, when it goes off, will generate a TimerEvent) More... | |
void | waitForAndProcessNextEvent () |
Wait for a workflow execution event and then call the associated function to process that event. | |
bool | waitForAndProcessNextEvent (double timeout) |
Wait for a workflow execution event and then call the associated function to process that event. More... | |
std::shared_ptr< WorkflowExecutionEvent > | waitForNextEvent () |
Wait for a workflow execution event. More... | |
std::shared_ptr< WorkflowExecutionEvent > | waitForNextEvent (double timeout) |
Wait for a workflow execution event. More... | |
Detailed Description
A workflow management system (WMS)
Constructor & Destructor Documentation
◆ WMS()
|
protected |
Constructor: a WMS with a workflow instance, a scheduler implementation, and a list of compute services.
- Parameters
-
standard_job_scheduler a standard job scheduler implementation (if nullptr then none is used) pilot_job_scheduler a pilot job scheduler implementation (if nullptr then none is used) compute_services a set of compute services available to run jobs (if {} then none is available) storage_services a set of storage services available to the WMS (if {} then none is available) network_proximity_services a set of network proximity services available to the WMS (if {} then none is available) file_registry_service a file registry services available to the WMS (if nullptr then none is available) hostname the name of the host on which to run the WMS suffix a string to append to the WMS process name (useful for debug output)
- Exceptions
-
std::invalid_argument
Member Function Documentation
◆ addDynamicOptimization()
void wrench::WMS::addDynamicOptimization | ( | std::unique_ptr< DynamicOptimization > | optimization | ) |
Add a dynamic optimization to the list of optimizations. Optimizations are executed in order of insertion.
- Parameters
-
optimization a dynamic optimization implementation
◆ addStaticOptimization()
void wrench::WMS::addStaticOptimization | ( | std::unique_ptr< StaticOptimization > | optimization | ) |
Add a static optimization to the list of optimizations. Optimizations are executed in order of insertion.
- Parameters
-
optimization a static optimization implementation
◆ addWorkflow()
void wrench::WMS::addWorkflow | ( | Workflow * | workflow, |
double | start_time = 0 |
||
) |
Assign a workflow to the WMS.
- Parameters
-
workflow a workflow to execute start_time the simulated time when the WMS should start executed the workflow (0 if not specified)
- Exceptions
-
std::invalid_argument
Set the simulation pointer member variable of the workflow so that the workflow tasks have access to it for creating SimulationTimestamps
◆ checkDeferredStart()
|
protected |
◆ createDataMovementManager()
|
protected |
Instantiate and start a data movement manager.
- Returns
- a data movement manager
◆ createEnergyMeter() [1/2]
|
protected |
Instantiate and start an energy meter.
- Parameters
-
measurement_periods the measurement period for each metered host
- Returns
- an energy meter
◆ createEnergyMeter() [2/2]
|
protected |
Instantiate and start an energy meter.
- Parameters
-
hostnames the list of metered hosts, as hostnames measurement_period the measurement period
- Returns
- an energy meter
◆ createJobManager()
|
protected |
Instantiate and start a job manager.
- Returns
- a job manager
◆ getAvailableComputeServices()
|
inlineprotected |
Obtain the list of compute services available to the WMS.
- Template Parameters
-
T ComputeService, or any if derived classes (but for CloudComputeService)
- Returns
- a set of compute services
◆ getAvailableFileRegistryService()
|
protected |
Obtain the file registry service available to the WMS.
- Returns
- a file registry services
◆ getAvailableNetworkProximityServices()
|
protected |
Obtain the list of network proximity services available to the WMS.
- Returns
- a set of network proximity services
◆ getAvailableStorageServices()
|
protected |
Obtain the list of storage services available to the WMS.
- Returns
- a set of storage services
◆ getPilotJobScheduler()
PilotJobScheduler * wrench::WMS::getPilotJobScheduler | ( | ) |
Get the WMS's pilot scheduler.
- Returns
- the pilot scheduler, or nullptr if none
◆ getStandardJobScheduler()
StandardJobScheduler * wrench::WMS::getStandardJobScheduler | ( | ) |
Get the WMS's pilot scheduler.
- Returns
- the pilot scheduler, or nullptr if none
◆ getWorkflow()
Workflow * wrench::WMS::getWorkflow | ( | ) |
Get the workflow that was assigned to the WMS.
- Returns
- a workflow
◆ processEventFileCopyCompletion()
|
protectedvirtual |
Process a file copy completion event.
- Parameters
-
event a FileCopyCompletedEvent
◆ processEventFileCopyFailure()
|
protectedvirtual |
Process a file copy failure event.
- Parameters
-
event a FileCopyFailedEvent
◆ processEventPilotJobExpiration()
|
protectedvirtual |
Process a pilot job expiration event.
- Parameters
-
event a PilotJobExpiredEvent
◆ processEventPilotJobStart()
|
protectedvirtual |
Process a pilot job start event.
- Parameters
-
event a PilotJobStartedEvent
◆ processEventStandardJobCompletion()
|
protectedvirtual |
Process a standard job completion event.
- Parameters
-
event a StandardJobCompletedEvent
◆ processEventStandardJobFailure()
|
protectedvirtual |
Process a standard job failure event.
- Parameters
-
event a StandardJobFailedEvent
◆ processEventTimer()
|
protectedvirtual |
Process a timer event.
- Parameters
-
event a TimerEvent
◆ setTimer()
|
protected |
Sets a timer (which, when it goes off, will generate a TimerEvent)
- Parameters
-
date the date at which the timer should go off message a string message that will be in the generated TimerEvent
◆ waitForAndProcessNextEvent()
|
protected |
Wait for a workflow execution event and then call the associated function to process that event.
- Parameters
-
timeout a timeout value in seconds
- Returns
- false if a timeout occurred (in which case no event was received/processed)
- Exceptions
-
wrench::WorkflowExecutionException
◆ waitForNextEvent() [1/2]
|
protected |
Wait for a workflow execution event.
- Returns
- the event
◆ waitForNextEvent() [2/2]
|
protected |
Wait for a workflow execution event.
- Parameters
-
timeout a timeout value in seconds
- Returns
- the event
The documentation for this class was generated from the following files:
- WMS.h
- WMS.cpp