A standard (i.e., non-pilot) workflow job that can be submitted to a ComputeService by a WMS (via a JobManager) More...
#include <StandardJob.h>
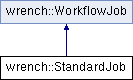
Public Types | |
enum | State { NOT_SUBMITTED, PENDING, RUNNING, COMPLETED, FAILED, TERMINATED } |
Standad job states. More... | |
![]() | |
enum | Type { STANDARD, PILOT } |
Job types. More... | |
Public Member Functions | |
std::map< WorkflowFile *, std::shared_ptr< FileLocation > > | getFileLocations () |
Get the file location map for the job. More... | |
unsigned long | getMinimumRequiredNumCores () |
Returns the minimum number of cores required, over all tasks in the job (i.e., at least one task in the job cannot run if fewer cores than this minimum are available) More... | |
unsigned long | getNumCompletedTasks () |
Get the number of completed tasks in the job. More... | |
unsigned long | getNumTasks () |
Get the number of tasks in the job. More... | |
unsigned long | getPriority () |
Get the workflow priority value (the maximum priority from all tasks) More... | |
StandardJob::State | getState () |
Get the state of the standard job. More... | |
std::vector< WorkflowTask * > | getTasks () |
Get the workflow tasks in the job. More... | |
![]() | |
std::string | getName () |
Get the job's name. More... | |
double | getSubmitDate () |
Get the date at which the job was last submitted (<0 means "never submitted") More... | |
Type | getType () |
Get the job type. More... | |
std::string | getTypeAsString () |
Get the job type name. More... | |
Public Attributes | |
std::vector< std::tuple< WorkflowFile *, std::shared_ptr< FileLocation > > > | cleanup_file_deletions |
The ordered file deletion operations to perform at the end. | |
std::map< WorkflowFile *, std::shared_ptr< FileLocation > > | file_locations |
The file locations that tasks should read/write files from/to. | |
unsigned long | num_completed_tasks |
The number of computational tasks that have completed. | |
std::vector< std::tuple< WorkflowFile *, std::shared_ptr< FileLocation >, std::shared_ptr< FileLocation > > > | post_file_copies |
The ordered file copy operations to perform after computational tasks. | |
std::vector< std::tuple< WorkflowFile *, std::shared_ptr< FileLocation >, std::shared_ptr< FileLocation > > > | pre_file_copies |
The ordered file copy operations to perform before computational tasks. | |
std::vector< WorkflowTask * > | tasks |
The job's computational tasks. | |
double | total_flops |
The job's total computational cost (in flops) | |
Detailed Description
A standard (i.e., non-pilot) workflow job that can be submitted to a ComputeService by a WMS (via a JobManager)
Member Enumeration Documentation
◆ State
Member Function Documentation
◆ getFileLocations()
std::map< WorkflowFile *, std::shared_ptr< FileLocation > > wrench::StandardJob::getFileLocations | ( | ) |
Get the file location map for the job.
- Returns
- a map of files to storage services
◆ getMinimumRequiredNumCores()
unsigned long wrench::StandardJob::getMinimumRequiredNumCores | ( | ) |
Returns the minimum number of cores required, over all tasks in the job (i.e., at least one task in the job cannot run if fewer cores than this minimum are available)
- Returns
- the number of cores
◆ getNumCompletedTasks()
unsigned long wrench::StandardJob::getNumCompletedTasks | ( | ) |
Get the number of completed tasks in the job.
- Returns
- the number of completed tasks
◆ getNumTasks()
unsigned long wrench::StandardJob::getNumTasks | ( | ) |
Get the number of tasks in the job.
- Returns
- the number of tasks
◆ getPriority()
unsigned long wrench::StandardJob::getPriority | ( | ) |
Get the workflow priority value (the maximum priority from all tasks)
- Returns
- the job priority value
◆ getState()
StandardJob::State wrench::StandardJob::getState | ( | ) |
Get the state of the standard job.
- Returns
- the state
◆ getTasks()
std::vector< WorkflowTask * > wrench::StandardJob::getTasks | ( | ) |
Get the workflow tasks in the job.
- Returns
- a vector of workflow tasks
The documentation for this class was generated from the following files:
- StandardJob.h
- StandardJob.cpp