A pilot (i.e., non-standard) workflow job that can be submitted to a ComputeService by a WMS (via a JobManager) More...
#include <PilotJob.h>
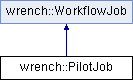
Public Types | |
enum | State { NOT_SUBMITTED, PENDING, RUNNING, EXPIRED, FAILED, TERMINATED } |
Pilot job states. More... | |
![]() | |
enum | Type { STANDARD, PILOT } |
Job types. More... | |
Public Member Functions | |
std::shared_ptr< ComputeService > | getComputeService () |
Get the compute service provided by the (running) pilot job. More... | |
unsigned long | getPriority () |
Get the pilot job priority value. More... | |
PilotJob::State | getState () |
Get the state of the pilot job. More... | |
void | setComputeService (std::shared_ptr< ComputeService > cs) |
Set the compute service on which the pilot job is running. More... | |
![]() | |
virtual | ~WorkflowJob () |
Destructor. | |
std::string | getCallbackMailbox () |
Get the "next" callback mailbox (returns the origin (i.e., workflow) mailbox if the mailbox stack is empty) More... | |
std::string | getName () |
Get the job's name. More... | |
std::string | getOriginCallbackMailbox () |
Get the "origin" callback mailbox. More... | |
std::shared_ptr< ComputeService > | getParentComputeService () |
Get the parent compute service of the job. More... | |
std::map< std::string, std::string > | getServiceSpecificArguments () |
Return the service-specific arguments that were used during job submission. More... | |
double | getSubmitDate () |
Get the date at which the job was last submitted (<0 means "never submitted") More... | |
Type | getType () |
Get the job type. More... | |
std::string | getTypeAsString () |
Get the job type name. More... | |
std::string | popCallbackMailbox () |
Get the "next" callback mailbox (returns the workflow mailbox if the mailbox stack is empty), and pops it. More... | |
void | pushCallbackMailbox (std::string) |
Pushes a callback mailbox. More... | |
void | setParentComputeService (std::shared_ptr< ComputeService > compute_service) |
Set the parent compute service of the job. More... | |
Additional Inherited Members | |
![]() | |
WorkflowJob (Type type) | |
Constructor. More... | |
unsigned long | getNewUniqueNumber () |
Generate a unique number (for each newly generated job) More... | |
![]() | |
std::stack< std::string > | callback_mailbox_stack |
Stack of callback mailboxes (to pop notifications) | |
std::string | name |
The job's name. | |
std::shared_ptr< ComputeService > | parent_compute_service |
The compute service to which the job was submitted. | |
std::map< std::string, std::string > | service_specific_args |
Service-specific arguments used during job submission. | |
double | submit_date |
The date at which the job was last submitted. | |
Type | type |
The job's type. | |
Workflow * | workflow |
The workflow this job belong to. | |
Detailed Description
A pilot (i.e., non-standard) workflow job that can be submitted to a ComputeService by a WMS (via a JobManager)
Member Enumeration Documentation
◆ State
Member Function Documentation
◆ getComputeService()
std::shared_ptr< ComputeService > wrench::PilotJob::getComputeService | ( | ) |
Get the compute service provided by the (running) pilot job.
- Returns
- a compute service
◆ getPriority()
|
virtual |
Get the pilot job priority value.
- Returns
- the pilot job priority value
Reimplemented from wrench::WorkflowJob.
◆ getState()
PilotJob::State wrench::PilotJob::getState | ( | ) |
Get the state of the pilot job.
- Returns
- the state
◆ setComputeService()
void wrench::PilotJob::setComputeService | ( | std::shared_ptr< ComputeService > | cs | ) |
Set the compute service on which the pilot job is running.
- Parameters
-
cs a compute service
The documentation for this class was generated from the following files:
- PilotJob.h
- PilotJob.cpp