Abstraction of a job used for executing tasks in a Workflow. More...
#include <WorkflowJob.h>
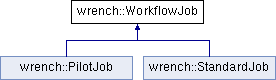
Public Types | |
enum | Type { STANDARD, PILOT } |
Job types. More... | |
Public Member Functions | |
virtual | ~WorkflowJob () |
Destructor. | |
std::string | getCallbackMailbox () |
Get the "next" callback mailbox (returns the origin (i.e., workflow) mailbox if the mailbox stack is empty) More... | |
std::string | getName () |
Get the job's name. More... | |
std::string | getOriginCallbackMailbox () |
Get the "origin" callback mailbox. More... | |
std::shared_ptr< ComputeService > | getParentComputeService () |
Get the parent compute service of the job. More... | |
virtual unsigned long | getPriority () |
Return default job priority as zero. More... | |
std::map< std::string, std::string > | getServiceSpecificArguments () |
Return the service-specific arguments that were used during job submission. More... | |
double | getSubmitDate () |
Get the date at which the job was last submitted (<0 means "never submitted") More... | |
Type | getType () |
Get the job type. More... | |
std::string | getTypeAsString () |
Get the job type name. More... | |
std::string | popCallbackMailbox () |
Get the "next" callback mailbox (returns the workflow mailbox if the mailbox stack is empty), and pops it. More... | |
void | pushCallbackMailbox (std::string) |
Pushes a callback mailbox. More... | |
void | setParentComputeService (std::shared_ptr< ComputeService > compute_service) |
Set the parent compute service of the job. More... | |
Protected Member Functions | |
WorkflowJob (Type type) | |
Constructor. More... | |
unsigned long | getNewUniqueNumber () |
Generate a unique number (for each newly generated job) More... | |
Protected Attributes | |
std::stack< std::string > | callback_mailbox_stack |
Stack of callback mailboxes (to pop notifications) | |
std::string | name |
The job's name. | |
std::shared_ptr< ComputeService > | parent_compute_service |
The compute service to which the job was submitted. | |
std::map< std::string, std::string > | service_specific_args |
Service-specific arguments used during job submission. | |
double | submit_date |
The date at which the job was last submitted. | |
Type | type |
The job's type. | |
Workflow * | workflow |
The workflow this job belong to. | |
Detailed Description
Abstraction of a job used for executing tasks in a Workflow.
Member Enumeration Documentation
◆ Type
Job types.
Enumerator | |
---|---|
STANDARD | A standard job that can be submitted directly to a ComputeService for execution. |
PILOT | A pilot job that can be submitted to a ComputeService and that, once started, will act as a ComputeService (likely a BareMetalComputeService) with an expiration date. |
Constructor & Destructor Documentation
◆ WorkflowJob()
|
protected |
Constructor.
- Parameters
-
type job type
Member Function Documentation
◆ getCallbackMailbox()
std::string wrench::WorkflowJob::getCallbackMailbox | ( | ) |
Get the "next" callback mailbox (returns the origin (i.e., workflow) mailbox if the mailbox stack is empty)
- Returns
- the next callback mailbox
◆ getName()
std::string wrench::WorkflowJob::getName | ( | ) |
Get the job's name.
- Returns
- the name as a string
◆ getNewUniqueNumber()
|
protected |
Generate a unique number (for each newly generated job)
- Returns
- a unique number
◆ getOriginCallbackMailbox()
std::string wrench::WorkflowJob::getOriginCallbackMailbox | ( | ) |
Get the "origin" callback mailbox.
- Returns
- the next callback mailbox
◆ getParentComputeService()
std::shared_ptr< ComputeService > wrench::WorkflowJob::getParentComputeService | ( | ) |
Get the parent compute service of the job.
- Returns
- a compute service
◆ getPriority()
|
virtual |
Return default job priority as zero.
- Returns
- priority as zero
Reimplemented in wrench::StandardJob, and wrench::PilotJob.
◆ getServiceSpecificArguments()
std::map< std::string, std::string > wrench::WorkflowJob::getServiceSpecificArguments | ( | ) |
Return the service-specific arguments that were used during job submission.
- Returns
- a map of argument name/values
◆ getSubmitDate()
double wrench::WorkflowJob::getSubmitDate | ( | ) |
Get the date at which the job was last submitted (<0 means "never submitted")
- Returns
- the submit date
◆ getType()
WorkflowJob::Type wrench::WorkflowJob::getType | ( | ) |
Get the job type.
- Returns
- the type
◆ getTypeAsString()
std::string wrench::WorkflowJob::getTypeAsString | ( | ) |
Get the job type name.
- Returns
- the type name as a string
- Exceptions
-
std::runtime_error
◆ popCallbackMailbox()
std::string wrench::WorkflowJob::popCallbackMailbox | ( | ) |
Get the "next" callback mailbox (returns the workflow mailbox if the mailbox stack is empty), and pops it.
- Returns
- the next callback mailbox
◆ pushCallbackMailbox()
void wrench::WorkflowJob::pushCallbackMailbox | ( | std::string | mailbox | ) |
Pushes a callback mailbox.
- Parameters
-
mailbox the mailbox name
◆ setParentComputeService()
void wrench::WorkflowJob::setParentComputeService | ( | std::shared_ptr< ComputeService > | compute_service | ) |
Set the parent compute service of the job.
- Parameters
-
compute_service a compute service
The documentation for this class was generated from the following files:
- WorkflowJob.h
- WorkflowJob.cpp