The compute service base class. More...
#include <ComputeService.h>
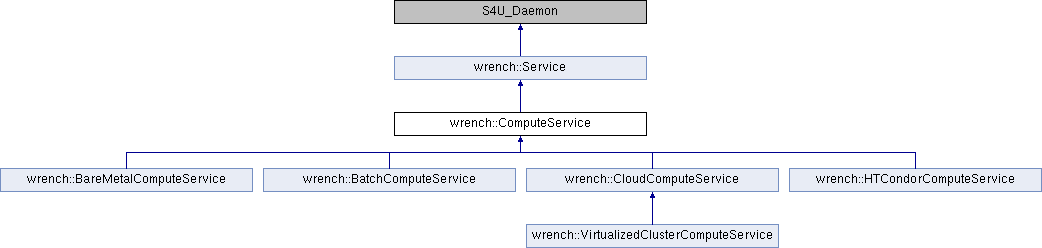
Public Member Functions | |
std::map< std::string, double > | getCoreFlopRate () |
Get the per-core flop rate of the compute service's hosts. More... | |
double | getFreeScratchSpaceSize () |
Get the free space on the compute service's scratch storage space. More... | |
std::vector< std::string > | getHosts () |
Get the list of the compute service's compute host. More... | |
std::map< std::string, double > | getMemoryCapacity () |
Get the RAM capacities for each of the compute service's hosts. More... | |
unsigned long | getNumHosts () |
Get the number of hosts that the compute service manages. More... | |
std::map< std::string, double > | getPerHostAvailableMemoryCapacity () |
Get ram availability for each of the compute service's host. More... | |
std::map< std::string, unsigned long > | getPerHostNumCores () |
Get core counts for each of the compute service's host. More... | |
std::map< std::string, unsigned long > | getPerHostNumIdleCores () |
Get idle core counts for each of the compute service's host. More... | |
std::shared_ptr< StorageService > | getScratchSharedPtr () |
Get a shared pointer to the compute service's scratch storage space. More... | |
unsigned long | getTotalNumCores () |
Get the total core counts for all hosts of the compute service. More... | |
virtual unsigned long | getTotalNumIdleCores () |
Get the total idle core count for all hosts of the compute service. More... | |
double | getTotalScratchSpaceSize () |
Get the total capacity of the compute service's scratch storage space. More... | |
double | getTTL () |
Get the time-to-live of the compute service. More... | |
bool | hasScratch () |
Checks if the compute service has a scratch space. More... | |
void | stop () override |
Stop the compute service - must be called by the stop() method of derived classes. | |
bool | supportsPilotJobs () |
Get whether the compute service supports pilot jobs or not. More... | |
bool | supportsStandardJobs () |
Get whether the compute service supports standard jobs or not. More... | |
void | terminateJob (std::shared_ptr< WorkflowJob > job) |
Terminate a previously-submitted job (which may or may not be running yet) More... | |
![]() | |
void | assertServiceIsUp () |
Throws an exception if the service is not up. More... | |
std::string | getHostname () |
Get the name of the host on which the service is / will be running. More... | |
double | getNetworkTimeoutValue () |
Returns the service's network timeout value. More... | |
bool | getPropertyValueAsBoolean (std::string) |
Get a property of the Service as a boolean. More... | |
double | getPropertyValueAsDouble (std::string) |
Get a property of the Service as a double. More... | |
std::string | getPropertyValueAsString (std::string) |
Get a property of the Service as a string. More... | |
unsigned long | getPropertyValueAsUnsignedLong (std::string) |
Get a property of the Service as an unsigned long. More... | |
bool | isUp () |
Returns true if the service is UP, false otherwise. More... | |
void | resume () |
Resume the service. More... | |
void | setNetworkTimeoutValue (double value) |
Sets the service's network timeout value. More... | |
void | start (std::shared_ptr< Service > this_service, bool daemonize, bool auto_restart) |
Start the service. More... | |
void | suspend () |
Suspend the service. | |
Static Public Attributes | |
static constexpr unsigned long | ALL_CORES = ULONG_MAX |
A convenient constant to mean "use all cores of a physical host" whenever a number of cores is needed when instantiating compute services. | |
static constexpr double | ALL_RAM = DBL_MAX |
A convenient constant to mean "use all ram of a physical host" whenever a ram capacity is needed when instantiating compute services. | |
Detailed Description
The compute service base class.
Member Function Documentation
◆ getCoreFlopRate()
std::map< std::string, double > wrench::ComputeService::getCoreFlopRate | ( | ) |
Get the per-core flop rate of the compute service's hosts.
- Returns
- a list of flop rates in flop/sec
- Exceptions
-
WorkflowExecutionException
◆ getFreeScratchSpaceSize()
double wrench::ComputeService::getFreeScratchSpaceSize | ( | ) |
Get the free space on the compute service's scratch storage space.
- Returns
- a size (in bytes)
◆ getHosts()
std::vector< std::string > wrench::ComputeService::getHosts | ( | ) |
Get the list of the compute service's compute host.
- Returns
- a vector of hostnames
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getMemoryCapacity()
std::map< std::string, double > wrench::ComputeService::getMemoryCapacity | ( | ) |
Get the RAM capacities for each of the compute service's hosts.
- Returns
- a map of RAM capacities, indexed by hostname
- Exceptions
-
WorkflowExecutionException
◆ getNumHosts()
unsigned long wrench::ComputeService::getNumHosts | ( | ) |
Get the number of hosts that the compute service manages.
- Returns
- the host count
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getPerHostAvailableMemoryCapacity()
std::map< std::string, double > wrench::ComputeService::getPerHostAvailableMemoryCapacity | ( | ) |
Get ram availability for each of the compute service's host.
- Returns
- the ram availability map (could be empty)
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getPerHostNumCores()
std::map< std::string, unsigned long > wrench::ComputeService::getPerHostNumCores | ( | ) |
Get core counts for each of the compute service's host.
- Returns
- a map of core counts, indexed by hostnames
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getPerHostNumIdleCores()
std::map< std::string, unsigned long > wrench::ComputeService::getPerHostNumIdleCores | ( | ) |
Get idle core counts for each of the compute service's host.
- Returns
- the idle core counts (could be empty)
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getScratchSharedPtr()
std::shared_ptr< StorageService > wrench::ComputeService::getScratchSharedPtr | ( | ) |
Get a shared pointer to the compute service's scratch storage space.
- Returns
- a shared pointer to the shared scratch space
◆ getTotalNumCores()
unsigned long wrench::ComputeService::getTotalNumCores | ( | ) |
Get the total core counts for all hosts of the compute service.
- Returns
- total core counts
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getTotalNumIdleCores()
|
virtual |
Get the total idle core count for all hosts of the compute service.
- Returns
- total idle core count
- Exceptions
-
WorkflowExecutionException std::runtime_error
◆ getTotalScratchSpaceSize()
double wrench::ComputeService::getTotalScratchSpaceSize | ( | ) |
Get the total capacity of the compute service's scratch storage space.
- Returns
- a size (in bytes)
◆ getTTL()
double wrench::ComputeService::getTTL | ( | ) |
Get the time-to-live of the compute service.
- Returns
- the ttl in seconds
- Exceptions
-
WorkflowExecutionException
◆ hasScratch()
bool wrench::ComputeService::hasScratch | ( | ) |
Checks if the compute service has a scratch space.
- Returns
- true if the compute service has some scratch storage space, false otherwise
◆ supportsPilotJobs()
bool wrench::ComputeService::supportsPilotJobs | ( | ) |
Get whether the compute service supports pilot jobs or not.
- Returns
- true or false
◆ supportsStandardJobs()
bool wrench::ComputeService::supportsStandardJobs | ( | ) |
Get whether the compute service supports standard jobs or not.
- Returns
- true or false
◆ terminateJob()
void wrench::ComputeService::terminateJob | ( | std::shared_ptr< WorkflowJob > | job | ) |
Terminate a previously-submitted job (which may or may not be running yet)
- Parameters
-
job the job to terminate
- Exceptions
-
std::invalid_argument WorkflowExecutionException std::runtime_error
The documentation for this class was generated from the following files:
- ComputeService.h
- ComputeService.cpp